The default stock status messages in WooCommerce aren’t as informative for customers as I’d like. If stock status messages are informative, they can help improve customers’ long-term shopping experience.
To solve the stock status message issue, I’ve written this simple guide. Let me show you how you can change and customize any of the “out of stock” or “in stock” messages with PHP snippets.
What is A PHP Snippet?
Basically, a PHP snippet is a small piece of reusable code that can provide additional functionality. PHP snippets can be pasted to different website content blocks, and they can also be considered as alternatives to plugins or modules.
Changing “Out of stock” Text with A Snippet
Simple products will have the default “out of stock” message. It’s really easy to change this to display another message, such as sold out or anything else. We need to access the woocommerce_get_availability_text
filter to change the availability message. We can also change the available stock message.
Advanced users can tap into the product details and create unique messages based on any conditions. For example, one condition could be if the product is in a specific category or not. I’ll include some bonus product conditions at the bottom for you to mix and match.
/**
* Change "Out of stock" to "Sold Out"
* @param string $text
* @param WC_Product $product
* @return string
*/
function puri_woocommerce_get_availability_text( $text, $product ) {
if (!$product->is_in_stock()) {
$text = 'Sold out';
} else {
// You can add more conditions here. e.g if product is available.
// $text = 'Available right now';
}
return $text;
}
add_filter( 'woocommerce_get_availability_text', 'puri_woocommerce_get_availability_text', 999, 2);
Code language: PHP (php)
Changing “product is currently out of stock” Text on Variable Products
Variable products have the default out of stock message “This product is currently out of stock and unavailable.” The WooCommerce filter we need to change is woocommerce_out_of_stock_message
. Let’s change it via PHP functions.
You can add the below function to your child theme’s functions.php or a custom plugin.
/**
* Out of stock message for product variations.
* @param string $text default message.
* @return string
*/
function puri_out_of_stock_message( $text ){
$text = 'This product is currently sold out, check back again soon.';
return $text;
}
add_filter( 'woocommerce_out_of_stock_message', 'puri_out_of_stock_message', 999);
Code language: PHP (php)
You can replace the default message with your own text. For example, “This product is currently sold out, check back again soon” could be a little more inviting.
Conditional WooCommerce “out of stock” Messages
You can conditionally apply a specific stock message per product or product category.
Here are a couple of examples to get you started. These snippets can be used within the above functions.
// Assuming you have access to the $product object.
// If the product has category
if( !has_term( 'uncategorized', 'product_cat', $product->get_id() )){
$text = 'uncategorized products are restocked every friday';
}
// If the product is a specific product by ID.
if( $product->get_id() == '123' )){
$text = 'This product is only restocked every friday';
}
Code language: PHP (php)
Custom Stock Status – The Plugin Alternative
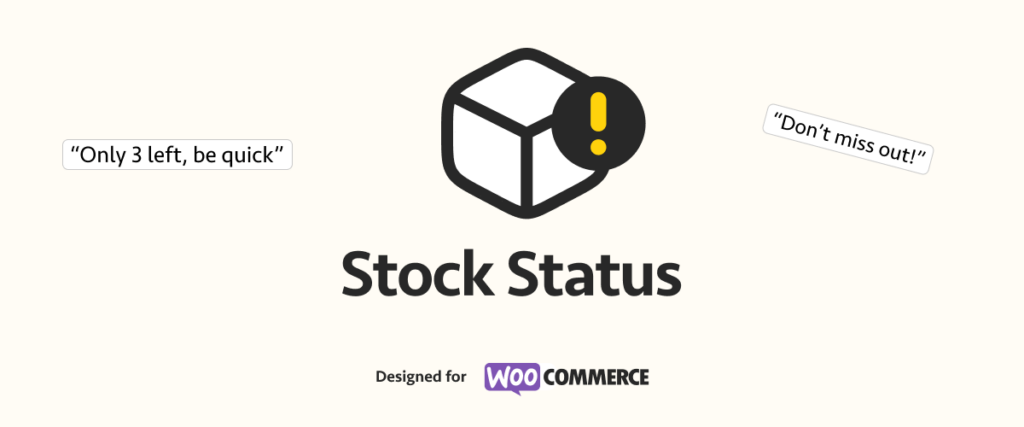
If you don’t want to tinker with snippets, you can just use the Custom Stock Status plugin. This plugin will let you set and customize stock status messages within the WooCommerce Settings panel.
You can use Custom Stock Status to customize stock messages of simple products. Additionally, the plugin also allows stock message editing of product variations. Plus, Custom Stock Status will allow you to set up Dynamic Date tags to inform customers whenever your products are back in stock.
Wrapping up
These snippets are fully compatible with WooCommerce 3.0 and WooCommerce 5.9+
I highly recommend adding these code snippets to your themes function.php file or using a plugin like Code Snippets. Also, feel free to read our guide about customizing your WooCommerce store with snippets.
If you looking for more ways to optimize stock handling in WooCommerce you should have a look at your plugins (Reserved Stock Pro & Stock Editor for WooCommerce) as well as more WooCommerce related articles on our blog.
Hi,
I copied and pasted the first bit of code into Snippets but no changes on my product page.
What is the exact code for changing the “in stock” text please?
Hey,
im just learning about hooks and filters. When looking through the documentation/code reference for woocommerce i cannot find anything called “woocommerce_out_of_stock_message”. Did the name change? Can’t find anything like it either.
Hi Alexander! When in doubt double check the core code directly on Github 😄 Here’s the woocommerce_out_of_stock_message