I’ve recently been diving deep into the WooCommerce core code to learn about updating stock via PHP functions and the REST API.
Therefore I thought I’d share a few pointers on both methods with my fellow developers!
1. Updating using WooCommerce functions
I will encourage you to use the official function wc_update_product_stock
. rather than some of the solutions, you’ll find on StackOverflow.
The wc_update_product_stock
function is also widely used within the WooCommerce
Tip: Don’t set stock metadata yourself. You risk running into issues. Let WooCommerce handle the metadata.
$product_id = 200;
$stock_quantity = 10;
wc_update_product_stock($product_id, $stock_quantity);
Code language: PHP (php)
The mentioned function is available in WooCommerce 3.0+ and the latest WooCommerce 5.9 at the time of writing.
2. Getting started with the WooCommerce REST API
The REST API is built into WooCommerce itself, it’s great for interacting with a WooCommerce store from a third-party app or even from within WordPress itself.
There are a few things you should consider before using the REST API.
Using the REST API within the WordPress admin dashboard is automatically authenticated and secure. You will not need to use API keys.
However, if you plan on using the WooCommerce REST API in a third-party app, you’ll need to generate API keys with “Read/Write” permissions.
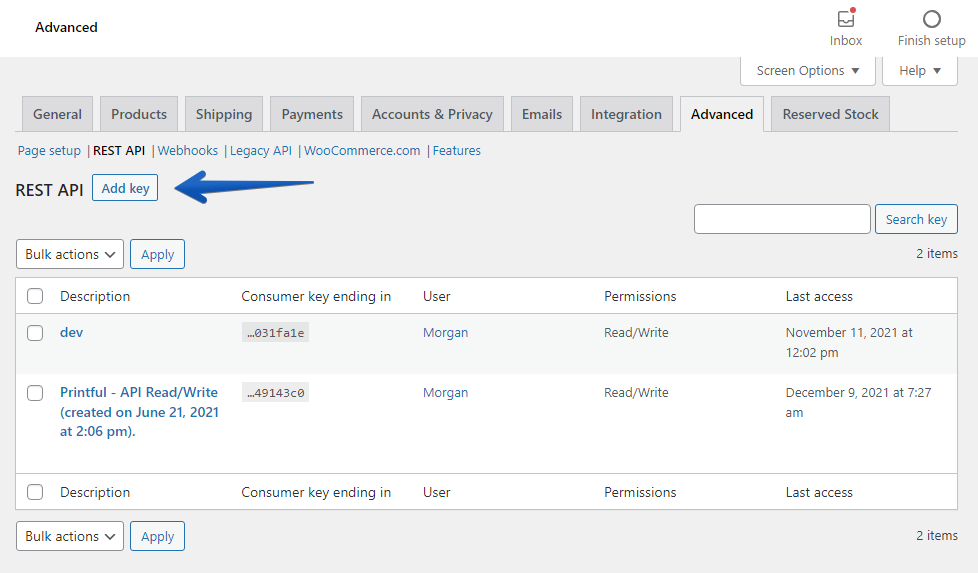
Remember – you will need to keep the API keys secure!
The keys provide full access to your store and customer data. If you do not take extra care of your API keys, then the hackers will sneak and snatch up everything.
Updating stock quantities with the REST API
Now that you’ve been warned and securely connected to the REST API, let us update some products!
Updating products is already documented in the official docs, so I’ll provide you with the simplified version using JavaScript.
Pure JavaScript with API ( with API Keys)
My example will assume you are using environment variables to store the WooCommerce public & secret keys.
const productId = "200";
const updatedData = { stock_quantity: 10 };
const options = {
method: "PUT",
headers: basicAuthHeaders(
process.env.WC_PUBLIC_KEY,
process.env.WC_SECRET_KEY
),
body: JSON.stringify(updatedData),
};
fetch("https://purirocks.com/wc/v3/products/" + productId, options)
.then((response) => response.json())
.then((data) => console.log(data));
Code language: JavaScript (javascript)
React & ApiFetch (without API Keys)
If you are using React and the WordPress scripts then I recommend looking at ApiFetch
. You can find more information on ApiFetch here.
You can use the method without API keys,
const productId = '200';
const updatedData = { stock_quantity: 10};
apiFetch({
path: "/wc/v3/products/" + productId,
method: "PUT",
data: updatedData,
}).then( ( res ) => {
console.log( res );
} );
Code language: JavaScript (javascript)
The response is should the freshly updated product object. You should check that the product did update as intended.
Wrapping up
Wait! Before you start coding the next stock management tool for your client. Save your precious time – I’ve already built the tool! Check out our Stock Editor for WooCommerce.
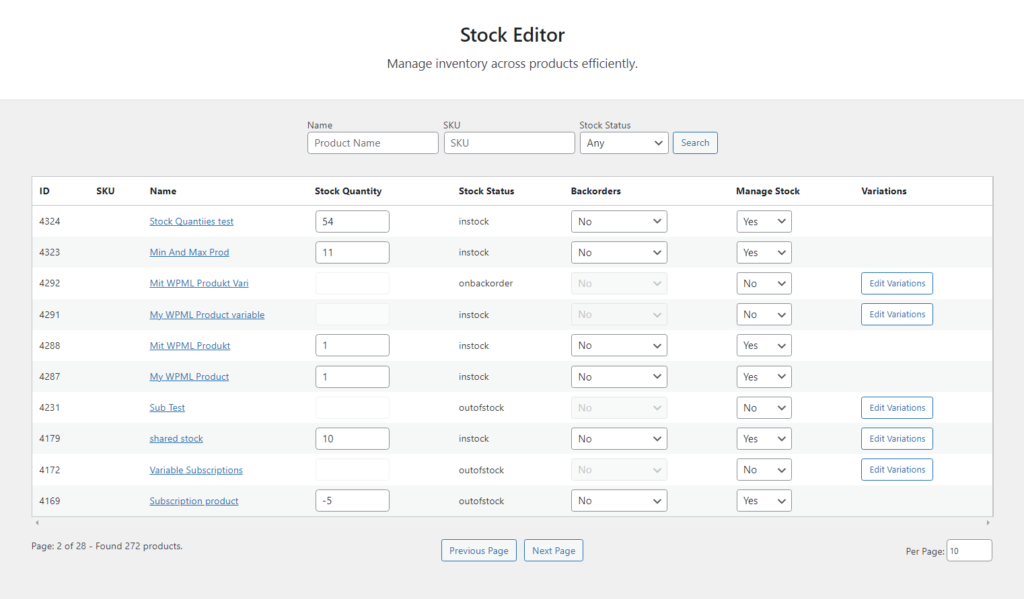
The Stock Editor is one of the fastest ways to update stock across all products and variations. We built the plugin to be super-fast, secure within the WordPress admin.
You and your client will love it.