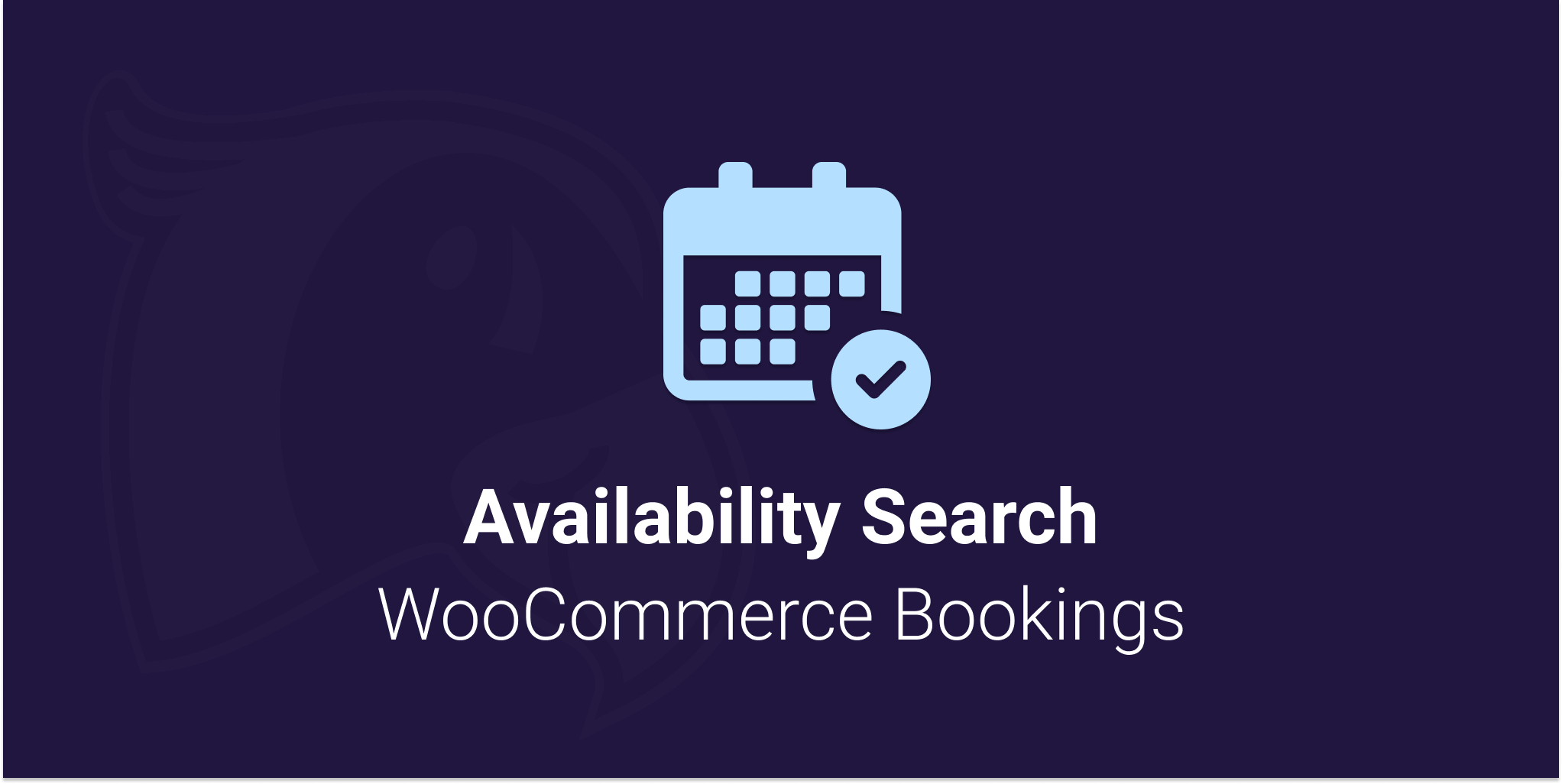
Viewing documentation for the Availability Search WooCommerce Bookings Plugin
Viewing documentation for the Availability Search WooCommerce Bookings Plugin
Developer level documentation. This is an advanced guide to customize the product loop. PHP knowledge is needed for a correct implementation.
The Availability Search for WooCommerce Bookings will use your themes’s product layout by default. We do recommend keeping the default layout for the best compatibility. However, if you do need to create your own, there’s a few examples on this page to help you.
Elementor is great way to quickly create a new product loop visually. Luckily Elementor now supports creating your own product loops for WooCommerce.
5342
ID in the below template and add the snippet to your site.Note: Modify your CSS might be required when using Elementor template.
function custom_aswb_loop_item( $product_id, $aswb_query ) {
// A specific Elementor Template Loop. Replace the ID with your own.
$output = do_shortcode('[elementor-template id="5342"]');
return $output;
}
add_filter('aswb_display_products','custom_aswb_loop_item', 10, 2 );
Code language: PHP (php)
Our starter plugin is made for developers to quickly start creating their own product loop with code.
You can find here https://github.com/puri-io/availability-search-custom-product-display
Use our aswb_display_products filter to override the default product template which is used in the Availability Search.
Here’s a simple example which output the name of each product found during the search. You can design your own product look here, or use shortcodes from other plugins if needed.
/*
* Override the Availability Search for WooCommerce bookings product display
* This example is simple and doesn't include any product details.
*/
function aswb_custom_filter_product_display( $product_id, $aswb_query ) {
// Use $product_id to get the product object, then you can access any data you'd like.
$product = wc_get_product( $product_id );
// Anything you and here must be returned, so it can be used for each product. This will display each name of the products
$output = "<h3>$product->get_name()</h3>";
return $output;
}
add_filter('aswb_display_products','aswb_custom_filter_product_display', 10 2 );
Code language: PHP (php)
Make sure to first call the WooCommerce Product object like so:
$product = wc_get_product( $product_id );
Code language: PHP (php)
You can now access any of the product details by using the below functions.
// Get Product ID
$product->get_id();
// Get Product General Info
$product->get_type();
$product->get_name();
$product->get_slug();
$product->get_date_created();
$product->get_date_modified();
$product->get_status();
$product->get_featured();
$product->get_catalog_visibility();
$product->get_description();
$product->get_short_description();
$product->get_sku();
$product->get_menu_order();
$product->get_virtual();
get_permalink( $product->get_id() );
// Get Product Prices
$product->get_price();
$product->get_regular_price();
$product->get_sale_price();
$product->get_date_on_sale_from();
$product->get_date_on_sale_to();
$product->get_total_sales();
// Get Product Tax, Shipping & Stock
$product->get_tax_status();
$product->get_tax_class();
$product->get_manage_stock();
$product->get_stock_quantity();
$product->get_stock_status();
$product->get_backorders();
$product->get_sold_individually();
$product->get_purchase_note();
$product->get_shipping_class_id();
// Get Product Dimensions
$product->get_weight();
$product->get_length();
$product->get_width();
$product->get_height();
$product->get_dimensions();
// Get Linked Products
$product->get_upsell_ids();
$product->get_cross_sell_ids();
$product->get_parent_id();
// Get Product Variations
$product->get_attributes();
$product->get_default_attributes();
// Get Product Taxonomies
$product->get_categories();
$product->get_category_ids();
$product->get_tag_ids();
// Get Product Downloads
$product->get_downloads();
$product->get_download_expiry();
$product->get_downloadable();
$product->get_download_limit();
// Get Product Images
$product->get_image_id();
get_the_post_thumbnail_url( $product->get_id(), 'full' );
$product->get_gallery_image_ids();
// Get Product Reviews
$product->get_reviews_allowed();
$product->get_rating_counts();
$product->get_average_rating();
$product->get_review_count();
// Latest updates can be found at https://docs.woocommerce.com/wc-apidocs/class-WC_Product.htm
Code language: PHP (php)